1. AOP(Aspect Orient Programing)
- 객체 지향 소프트웨어 개발에서 횡단 관심사 또는 크로스커팅 관심사(cross-cutting concerns)는 다른 관심사에 영향을 미치는 프로그램의 애스펙트이다.
- 요구 사항에 대해 핵심 관심사항과 횡단 관심사항으로 분할, 개발, 통합하여 모듈화를 극대화하는 프로그램 기법
=> 결국, 횡단 관심 요소들의 처리를 모듈화를 통한 효율적으로 극복
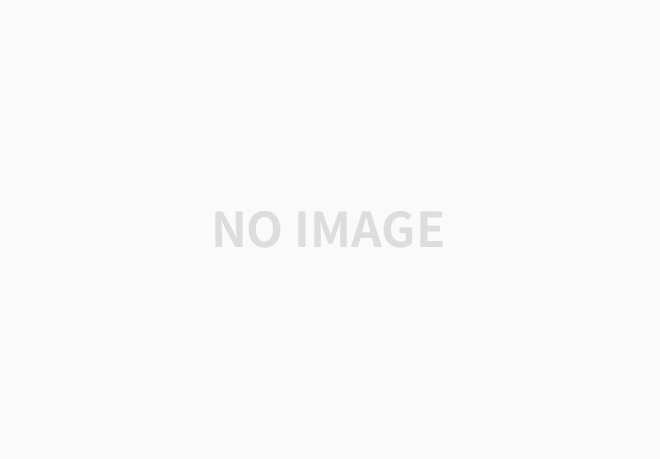
2. AOP의 구성요소
- 핵심 관심 : 시스템의 핵심 가치와 목적이 드러난 관심 영역
- 횡단 관심 : 쉽게 분리된 모듈로 작성하기 힘든 요구 사항, 공통 모듈
- Joint Point : 합류 지점, 메소드 실행시점
- Pointcut : 어디에 적용할 것인가, 계좌이체, 입출금, 이자 계산 적용대상
- Aspect : 모듈
- Advice : 해야할일
3. AOP 해보기(Spring Boot 사용)
- 패키지 목록
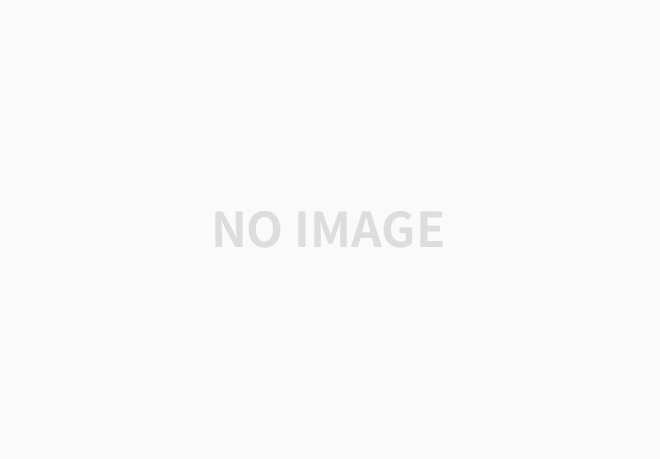
3-1. pom.xml에 aop 요소
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
3-2. Aop 적용할 커스텀 어노테이션 생성(AopAnnotation.java)
import java.lang.annotation.*;
/**
* 커스텀 어노테이션<br/>
* Documented : javadoc으로 문서 생성 시 현재 어노테이션 설명 추가 <br/>
* Retention : 어노테이션을 유지하는 정책 설정<br/>
* Target : 어노테이션 적용 위치
*/
@Documented
@Retention(RetentionPolicy.CLASS)
@Target(ElementType.METHOD)
public @interface AopAnnotation {
}
3-3. 테스트할 서비스(클래스) 작성(AopService.java)
import org.springframework.stereotype.Service;
@Service
public class AopService {
@AopAnnotation
public void print(){
System.out.println("Aop Service print문 실행");
}
@AopAnnotation
public int returnIntValue(){
System.out.println("returnIntValue 에서 값 10 return 합니다");
return 10;
}
}
3-4. AOP 클래스 생성(AopClass.java)
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Component
@Aspect
public class AopClass {
@Around("@annotation(AopAnnotation)")
public Object aopTest(ProceedingJoinPoint proceedingJoinPoint) throws Throwable{
System.out.println("AopClass aopTest @Around @annotation(AopAnnotation) 동작");
Object resultValue = proceedingJoinPoint.proceed();
System.out.println("resultValue = > " + resultValue);
return resultValue;
}
@Around("@annotation(AopAnnotation)")
public Object aopTest2(ProceedingJoinPoint proceedingJoinPoint) throws Throwable{
System.out.println("AopClass aopTest2 @Around @annotation(AopAnnotation) 동작");
Object resultValue = proceedingJoinPoint.proceed();
return resultValue;
}
}
3-5. AppRunner 작성 (AppRunner.java)
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.stereotype.Component;
@Component
public class AppRunner implements ApplicationRunner {
@Autowired
AopService aopService;
@Override
public void run(ApplicationArguments args) throws Exception {
aopService.print();
aopService.returnIntValue();
}
}
4. 결과화면
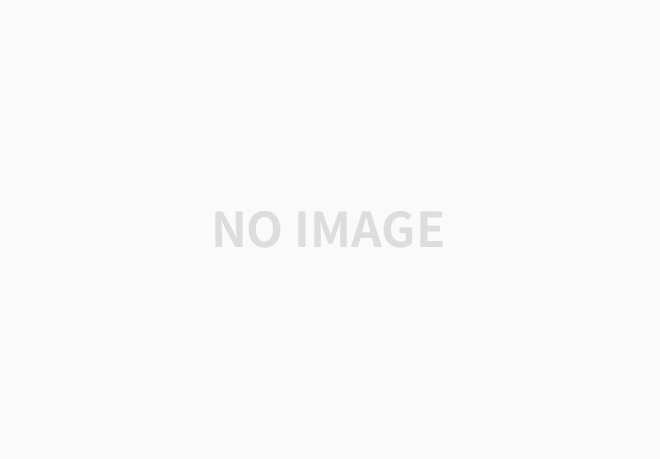
'[Spring]' 카테고리의 다른 글
@GetMapping 테스트 해보기 (0) | 2020.04.02 |
---|---|
[Spring 에러] intellij release version 5/11/12 not supported (0) | 2020.03.31 |
[Spring]Spring Validation 사용해보기 (0) | 2020.03.17 |
[Spring] Bytebuddy를 사용한 .class 바꾸기 (0) | 2020.03.15 |
[Spring기초] @Autowried , @Qualifier의 이해 (0) | 2019.10.25 |